You can use the scheduling in Cinema.jar to schedule macros daily, weekly or monthly. When Cinema.jar is installed and executed for the first time it will create 3 default keys. They will look like this in the registry.
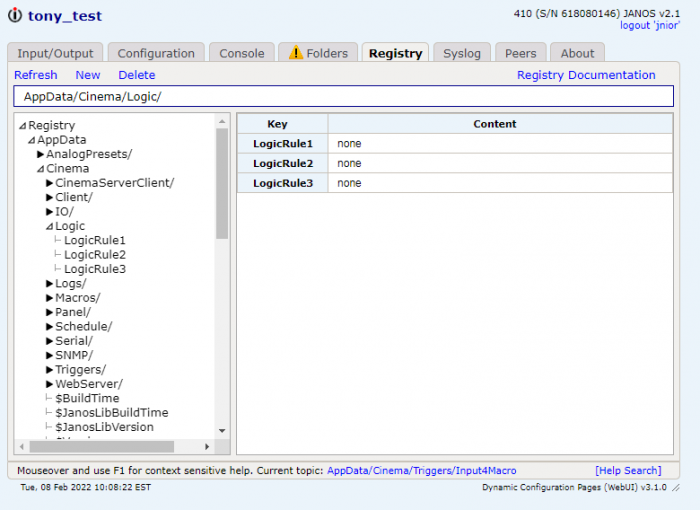
Below are examples for the logic you can implement in Cinema using these registries.
OnIOChange
This logic expression will be checked whenever the JNIOR I/O changes. The I/O that can be monitored are the internal inputs and outputs on the JNIOR as well as any outputs on a 4 relay output expansion module.
OnIOChange, din1 and din2, run test
For the above example, each time either input 1 or 2 changes, the logic rule (din1 AND din2) will be evaluated and the macro (test) will execute if both inputs are ON.
OnPreshowClient()
This logic expression will be checked whenever the Preshow Client in Cinema.JAR receives a message. To specify the string/message that will cause the logic to be evaluated put it inside the parenthesis.
OnPreshowClient(start), din3 and din4, run preshow start
For the above example, each time the device connected to the JNIOR as the Preshow Client sends the string “start” to Cinema.JAR, the logic rule (din3 AND din4) will be evaluated and the macro (preshow start) will execute if both inputs are ON.
OnCinemaServer()
This logic expression will be checked whenever the Cinema Server Client in Cinema.JAR receives a message. To specify the string/message that will cause the logic to be evaluated put it inside the parenthesis.
OnCinemaServer(movie), din5 or din6, run movie start
For the above example, each time the device connected to the JNIOR as the Cinema Server Client sends the string “movie”, the logic (din5 OR din6) will be evaluated and the macro (movie start) will be run if either digital input is ON.
OnMacro()
This logic expression will be evaluated whenever the named macro has been requested to run. This logic expression can be used as a ‘lock’ to make sure certain macros can only execute when a certain I/O state exists or cannot be executed when a certain I/O state exists. To specify the macro that is bound by this logic place its name inside the parenthesis.
OnMacro(flat start), din7
For the above example, if the macro “flat start” is triggered to run via any method, the logic (din7) will be checked. If (din7) is ON, the macro will be allowed to run. If (din7) is OFF, the macro will not be allowed to run.
Below is an example registry using some of the examples above.
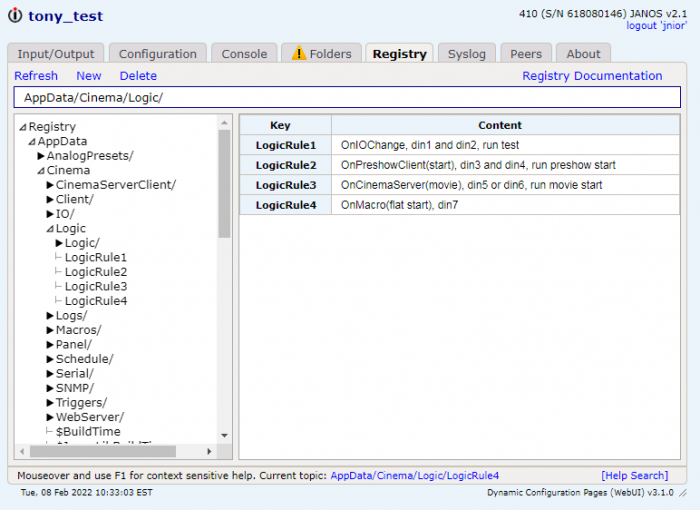